I have been in DevOps related jobs for past 6 years dealing mainly with Kubernetes in AWS and on-premise as well. I spent quite a lot …
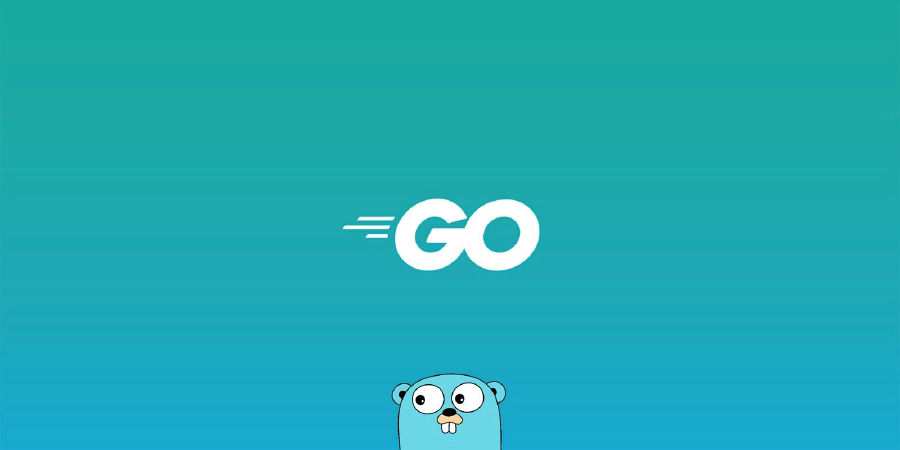
Go concurency
// package main
// import (
// "fmt"
// // "strconv"
// // "math"
// // "reflect"
// // "net/http"
// // "log"
// )
// // define interface
// type Writer interface {
// Write([]byte) (int, error)
// }
// type ConsoleWriter struct {}
// func (cw ConsoleWriter) Write(data []byte) (int, error) {
// n, err := fmt.Printf("Converts bytes to string: %v\n", string(data))
// return n, err
// }
// func main() {
// var w Writer = ConsoleWriter{}
// w.Write([]byte("101010100001"))
// }
package main
import (
"fmt"
"time"
)
func sayHello() {
fmt.Printf("Say hello\n")
}
func main() {
go sayHello()
time.Sleep(100 * time.Millisecond)
// bad approach
var msg string = "hello"
go func (i string) {
fmt.Printf("%v\n", i)
}(msg)
msg = "Goodbye"
time.Sleep(100 * time.Millisecond)
}