I have been in DevOps related jobs for past 6 years dealing mainly with Kubernetes in AWS and on-premise as well. I spent quite a lot …
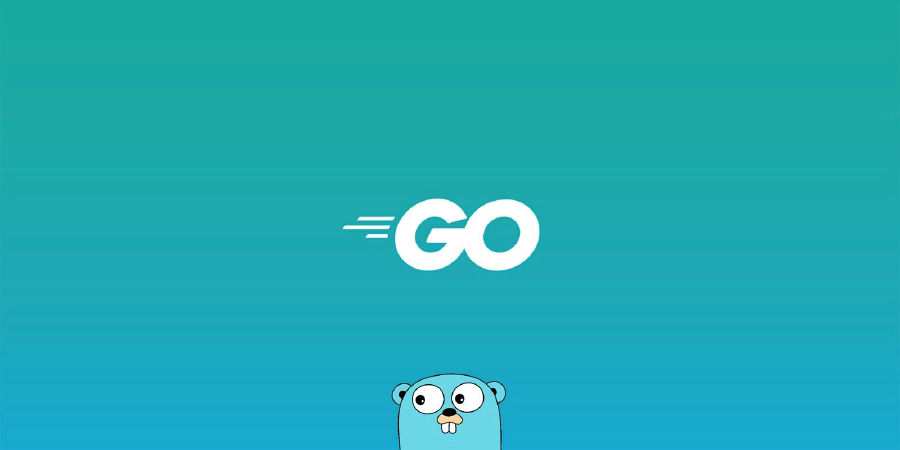
Go interfaces
// package main
// import (
// "fmt"
// // "strconv"
// // "math"
// // "reflect"
// // "net/http"
// // "log"
// )
// // define interface
// type Writer interface {
// Write([]byte) (int, error)
// }
// type ConsoleWriter struct {}
// func (cw ConsoleWriter) Write(data []byte) (int, error) {
// n, err := fmt.Printf("Converts bytes to string: %v\n", string(data))
// return n, err
// }
// func main() {
// var w Writer = ConsoleWriter{}
// w.Write([]byte("101010100001"))
// }
package main
import (
"fmt"
)
type Shape interface {
Area() float64
Perimeter() float64
}
type Rect struct {
width float64
hight float64
}
func (r Rect) Area() float64 {
return r.width * r.hight
}
func (r Rect) Perimeter() float64 {
return 2 * (r.width + r.hight)
}
func main() {
var s Shape = Rect{
width: 5.0,
hight: 4.0,
}
r := Rect{
width: 5.0,
hight: 4.0,
}
fmt.Printf("%v\n", s.Perimeter())
fmt.Printf("%v\n", s.Area())
fmt.Printf("%T\n", s)
fmt.Printf("%v\n", r.Perimeter())
fmt.Printf("%v\n", r.Area())
fmt.Printf("%T\n", r)
}