I have been in DevOps related jobs for past 6 years dealing mainly with Kubernetes in AWS and on-premise as well. I spent quite a lot …
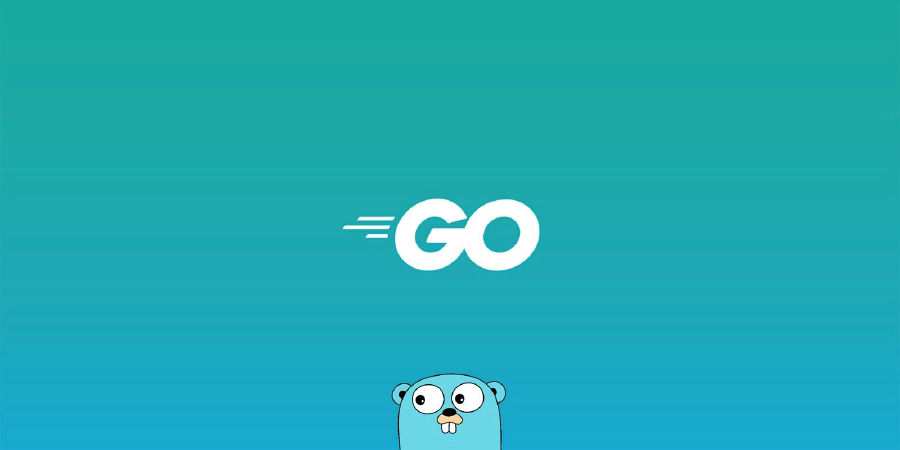
Go loop
package main
import (
"fmt"
// "strconv"
// "math"
// "reflect"
// "math"
)
func basicLoop() {
for i := 0; i < 5; i++ {
fmt.Printf("Number: %v\n", i)
}
}
func everyOther() {
for i := 0; i < 5; i = i + 2 {
fmt.Printf("Number: %v\n", i)
}
}
func loopOverTwoVariables() {
for i, j := 0, 0; i < 5; i, j = i + 1, j + 3 {
fmt.Printf("i: %v, j: %v\n", i, j)
}
}
func play() {
for i:= 0; i < 5; i++ {
fmt.Printf("Number: %v\n", i)
if i%2 == 0 {
i /= 2
} else {
i = 2*i + 1
}
}
}
func initializerAtSomeOtherPlace() {
i := 0
for ; i < 5; i++ {
fmt.Printf("Number: %v\n", i)
}
}
func pythonLike() {
i := 0
// for i < 5 { <- works just fine withour semicolons
for ; i < 5 ; {
fmt.Printf("Number: %v\n", i)
i++
}
}
func infiniteForLoop() {
i := 0
for {
fmt.Printf("Number: %v\n", i)
i++
}
}
func infiniteForLoopWithBreak() {
i := 0
for {
fmt.Printf("Number: %v\n", i)
i++
if i == 5 {
fmt.Printf("Breaking from Infinite Loop! number: %v\n", i)
break
}
}
}
func forLoopWithContinueKeyWork() {
for i := 0; i < 10; i ++ {
if i%2 == 0 {
// the keyword "continue" is a special keyword
// and if this condition above is true that we
// stop here and don't go and print anything !!!
// we sort of continuing back to main loop
continue
}
fmt.Printf("Number: %v\n", i)
}
}
func nestedForLoop() {
for i := 1; i <= 3; i++ {
for j := 1; j <=4; j++ {
fmt.Printf("Multiply %v(i) * %v(j) = %v\n", i, j, i*j)
}
}
}
func breakOutOfNestedForLoop() {
// This "label" below "tells to" the "break" keyword
// which loop to exit!!! Please notice that if this label not used
// only inner most loop would be exited
Labelloop:
for i := 1; i <= 3; i++ {
for j := 1; j <=4; j++ {
fmt.Printf("Multiply %v(i) * %v(j) = %v\n", i, j, i*j)
if i * j >= 3 {
fmt.Printf("Breaking out of nested for loop because of condition based on label!!!\n")
break Labelloop
}
}
}
}
func collectionForLoop() {
s := []int{1, 2, 3}
fmt.Printf("Element: %v\n", s)
fmt.Println("")
for k, v := range s {
fmt.Printf("Element: %v(k): %v(v)\n", k, v)
}
fmt.Println("")
// maps
statePopulation := make(map[string]int)
statePopulation = map[string]int{
"California": 2341232,
"Texas": 3341232,
"Florida": 4341232,
"New York": 5341232,
"Illinois": 6341232,
"Ohio": 7341232,
}
fmt.Println("")
for k,v := range statePopulation {
fmt.Printf("Element: %v(k): %v(v)\n", k, v)
}
fmt.Println()
// if we only care for "keys"
for k := range statePopulation {
fmt.Printf("Element: %v(k)\n", k)
}
fmt.Println("")
// if we only care for "values"
for _, v := range statePopulation {
fmt.Printf("Element: %v(v)\n", v)
}
fmt.Println("")
// strings
st := "hello Go!"
for k, v := range st {
fmt.Printf("Element: %v(k): %v(v), %v(v) \n", k, v, string(v))
}
}
func main() {
// basicLoop()
// everyOther()
// loopOverTwoVariables()
// play()
// initializerAtSomeOtherPlace()
// pythonLike()
// infiniteForLoop()
// infiniteForLoopWithBreak()
// forLoopWithContinueKeyWork()
// nestedForLoop()
// breakOutOfNestedForLoop()
collectionForLoop()
}