I have been in DevOps related jobs for past 6 years dealing mainly with Kubernetes in AWS and on-premise as well. I spent quite a lot …
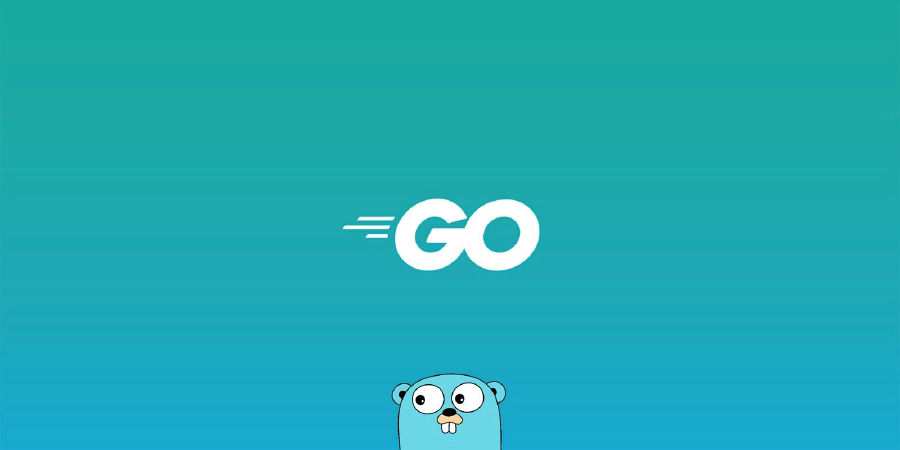
Go template hotels
package main
import (
"os"
"log"
"text/template"
)
type hotel struct {
Name string
Address string
City string
Zip []int
Region string
}
var tpl *template.Template
func init() {
tpl = template.Must(template.ParseFiles("tpl.gohtml"))
}
func main() {
hotelsList := []hotel{
hotel{
Name: "Hilton",
Address: "Atlantic Ave",
City: "Paulo Alto",
Zip: []int{2,3,4},
Region: "CAL1",
},
hotel{
Name: "M&M",
Address: "Pacific Ave",
City: "San Francisco",
Zip: []int{1,3,4},
Region: "CAL2",
},
hotel{
Name: "Labut",
Address: "Indian Ave",
City: "San Diego",
Zip: []int{2,1,4},
Region: "CAL3",
},
}
err := tpl.Execute(os.Stdout, hotelsList)
if err != nil {
log.Fatalln(err)
}
}
// *.gothml file
cat golang-web-dev/012_hands-on/03_hands-on/tpl.gohtml
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Composition</title>
</head>
<body>
{{range .}}
{{.Name}} - {{.City}} - {{.Zip}} - {{.Region}} - {{.Address}}
{{end}}
</body>
</html>%