I have been in DevOps related jobs for past 6 years dealing mainly with Kubernetes in AWS and on-premise as well. I spent quite a lot …
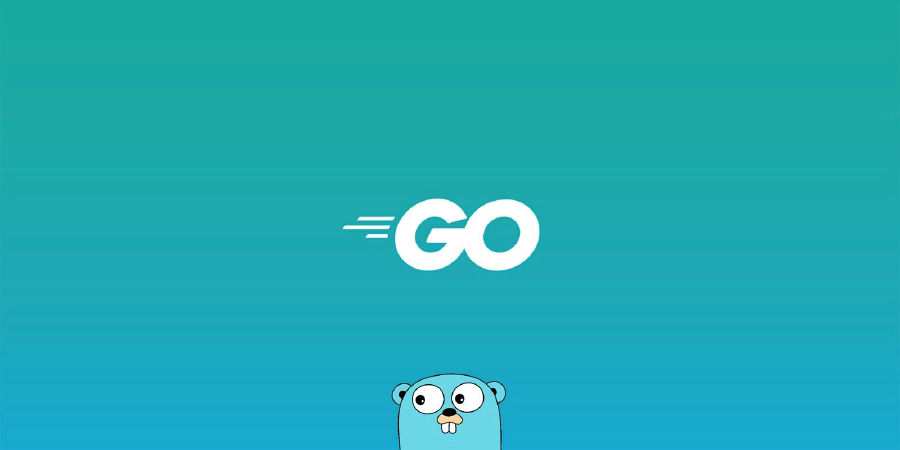
Go methods templates and composition
package main
import (
"log"
"os"
"text/template"
)
type person struct {
Name string
Age int
}
// Start -Let's define several methods for struct person
func (p person) SomeProcessing() int {
return 7
}
func (p person) AgeDbl() int {
return p.Age * 2
}
func (p person) TakesArg(x int) int {
return x * 2
}
// End - Let's define several methods for struct person
type doubleZero struct {
person
LicenseToKill bool
}
type course struct {
Number string
Name string
Units int
}
type semester struct {
Term string
Courses []course
}
type year struct {
Fall semester
Spring semester
Summer semester
}
var tpl *template.Template
func init() {
// tpl = template.Must(template.ParseFiles("neasted2.gohtml"))
tpl = template.Must(template.ParseFiles("methods.gohtml", "neasted2.gohtml"))
}
func main() {
p1 := person{
Name: "James Bond",
Age: 47,
}
y := year{
Fall: semester{
Term: "Fall",
Courses: []course{
course{
Number: "F01",
Name: "Introduction to Go Programmig",
Units: 3,
},
course{
Number: "F02",
Name: "Introduction to Python Programmig",
Units: 4,
},
course{
Number: "F03",
Name: "Introduction to Ruby Programmig",
Units: 5,
},
},
},
Spring: semester{
Term: "Spring",
Courses: []course{
course{
Number: "S01",
Name: "Introduction to Matlab Programmig",
Units: 8,
},
course{
Number: "S02",
Name: "Introduction to Lua Programmig",
Units: 7,
},
course{
Number: "S03",
Name: "Introduction to Java Programmig",
Units: 6,
},
},
},
Summer: semester{
Term: "Summer",
Courses: []course{
course{
Number: "Summer01",
Name: "Introduction to C++ Programmig",
Units: 8,
},
course{
Number: "Summer02",
Name: "Introduction to Delphi Programmig",
Units: 7,
},
course{
Number: "Summer03",
Name: "Introduction to Pascal Programmig",
Units: 6,
},
},
},
}
// err := tpl.Execute(os.Stdout, y)
err := tpl.ExecuteTemplate(os.Stdout, "neasted2.gohtml", y)
if err != nil {
log.Fatalln(err)
}
err = tpl.ExecuteTemplate(os.Stdout, "methods.gohtml", p1)
if err != nil {
log.Fatalln(err)
}
}
// file: neasted2.gohtml
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
{{range .Spring.Courses}}
Number: {{.Number}} - {{.Name}} - {{.Units}}
{{end}}
{{range .Fall.Courses}}
Number: {{.Number}} - {{.Name}} - {{.Units}}
{{end}}
{{range .Summer.Courses}}
Number: {{.Number}} - {{.Name}} - {{.Units}}
{{end}}
</body>
</html>
// methods.gohtml
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Composition</title>
</head>
<body>
<h1>{{.Name}}</h1>
<h2>{{.Age}}</h2>
<h3>{{.SomeProcessing}}</h3>
<h3>{{.AgeDbl}}</h3>
<h3>{{.Age | .TakesArg}}</h3>
<h3>{{.AgeDbl | .TakesArg}}</h3>
</body>
</html>